31
Jul
Joomla wordpress Integration
- Category:
- Joomla
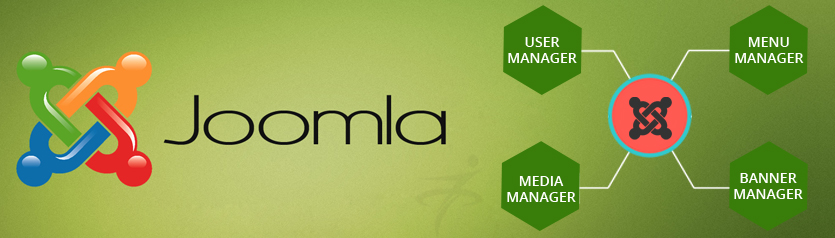
Posted On : July 31, 2013
| 1 Comment
Here we are using Joomla 2.5 and WordPress 3.5 .In this tutorial we will see how to do some basic integration of Joomla with WordPress.
Adding Article to both Joomla and WordPress from Joomla backend
In order to add article to wordpress from Joomla we have to add the data into the database of WordPress at the time of saving the article in Joomla. Articles will be inserted on the home page of wordpress.
Finding the location of save operation in Joomla
We have to add the code for adding the article to database of WordPress at the place where Joomla saves the Article.Joomla does this in file controllerform.php at the location “\libraries\joomla\application\component\” in function save().You can check it by adding some message at the end of this function.Add this code at the end of save() function to check it.
$app->enqueueMessage(‘I Am Here’, ‘error’);
You will get this message when you save the article.
View the database of wordpress
You can view the database of wordpress using phpMyAdmin. Now select the database you have created for wordpress, you will find there all the tables in the database.Here we will insert or update data in two tables:
1) #__posts – for saving all the information about articles.
2) #__term_relationships – for saving the article format as gallery.
View the database of joomla
You can view the database of joomla using phpMyAdmin. Now select the database you have created for joomla, you will find there all the tables in the database.Here we can see all the information about the article in following table:
1) #__content
Using the external database in joomla
To use the external database in Joomla we have to get database object by getInstance() function of JDatabase class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | $option = array(); $option['driver'] = 'mysql'; // Database driver name $option['host'] = 'localhost'; // Database host name $option['user'] = 'root'; // Database User Name $option['password'] = 'root'; // Database Password $option['database'] = 'wordpress'; // Database name to be accessed $option['prefix'] = 'table_'; // Database table name Prefix $dbw = & JDatabase::getInstance($option); |
For the Joomla database we use getDBO() function of JFactory class.
$dbj = & JFactory::getDBO();
Now we can use this database object to access the database.
Getting the information about article in joomla
We can get the form data by using the following command:
$data = JRequest::getVar(‘jform’, array(), ‘post’, ‘array’);
Now we will use this $data variable further in this article.
We can get all the information about the article by using this $data variable.
To get the title of the article use $data['title'],to get other information about the article you can give the column name of the table used by joomla database in place of ‘title’, dont forget to give it within single quote.
To get the article content don’t use the column name, instead of it use following command:
1 2 3 4 5 6 7 8 9 10 | $post_content = $data['articletext']; $dbw->setQuery( 'INSERT INTO #__posts ( ID, post_author, post_date, post_date_gmt, post_content, post_title, post_excerpt, post_status, comment_status, ping_status, post_password, post_name, to_ping, pinged, post_modified, post_modified_gmt, post_content_filtered, post_parent, guid, menu_order, post_type, post_mime_type, comment_count )'. ' VALUES (NULL , 1, '.$dbw->Quote($data['created']).', '.$dbw->Quote($data['created']).', '.$dbw->Quote($post_content).', '.$dbw->Quote($data['title']).','.$dbw->Quote('').','.$dbw->Quote('publish').','.$dbw->Quote('open').','.$dbw->Quote('open').','.$dbw->Quote('').','.$dbw->Quote($data['alias']).','.$dbw->Quote('').','.$dbw->Quote('').','.$dbw->Quote($data['modified']).','.$dbw->Quote($data['modified']).','.$dbw->Quote('').',0,'.$dbw->Quote('http://localhost/wordpress/?p=').','.$dbw->Quote($data['ordering']).','.$dbw->Quote('post').','.$dbw->Quote('').',0 )' ); if (!$dbw->query()) { $app->enqueueMessage("error in db saving ", 'warning'); return false; } |
wordpress table #__posts uses guid column to store the url for the article.The guid should look like the following http://localhost/wordpress/?p=56 Here “http://localhost/wordpress/” is the url of the wordpress.”56″ is the post ID, which is unique for each article in the #__posts table.
In the above written insert query we have stored guid value as “http://localhost/wordpress/?p=”.Now we will give the post id by select and the update query as shown below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | $queryw=$dbw->getQuery(true); $queryw->select('max(ID) AS id'); $queryw->from('#__posts'); $dbw->setQuery($queryw); $messagew=$dbw->loadObjectList(); $messagew[0]->id $dbw->setQuery( 'UPDATE #__posts SET guid = concat(guid,'.$messagew[0]->id.') where ID='.$messagew[0]->id.'' ); if (!$dbw->query()) { $app->enqueueMessage("error in update db" , 'warning'); return false; } 2) in table #__term_relationships We are inserting in this table to get "Continue reading" link in the article. Gallery format of the article will give the "Continue reading" link. $dbw->setQuery( 'INSERT INTO #__term_relationships ( object_id, term_taxonomy_id, term_order)'. ' VALUES ('.$messagew[0]->id.' , 4,0 )' ); if (!$dbw->query()) { $app->enqueueMessage("error in db saving in #__term_relationships" , 'warning'); return false; } |
Here “term_taxonomy_id” is set as “4″, 4 is the value for gallery format.
The full code would look like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 | public function saveWord() { $app = JFactory::getApplication(); $data = JRequest::getVar('jform', array(), 'post', 'array'); $option = array(); //prevent problems $option['driver'] = 'mysql'; // Database driver name $option['host'] = 'localhost'; // Database host name $option['user'] = 'root'; // User for database authentication $option['password'] = 'root'; // Password for database authentication $option['database'] = 'wordpress'; // Database name $option['prefix'] = 'table_'; // Database prefix (may be empty) $dbw = & JDatabase::getInstance($option); $queryw=$dbw->getQuery(true); $post_content = $data['articletext']; $titleToComp = $data['title']; $queryw=$dbw->getQuery(true); $queryw->select('ID'); $queryw->from('#__posts'); $queryw->where('post_title ='. $dbw->Quote($titleToComp).''); $dbw->setQuery($queryw); $messagew1=$dbw->loadObjectList(); if(count($messagew1) > 0) { $dbw->setQuery( 'UPDATE #__posts SET post_content = '.$dbw->Quote($post_content).' where ID='.$messagew1[0]->ID.'' ); if (!$dbw->query()) { $app->enqueueMessage("error in update db " , 'warning'); return false; } $app->enqueueMessage("Article updated to wordpress with article ID ".$messagew1[0]->ID , 'message'); } else { $dbw->setQuery( 'INSERT INTO #__posts ( ID, post_author, post_date, post_date_gmt, post_content, post_title, post_excerpt, post_status, comment_status, ping_status, post_password, post_name, to_ping, pinged, post_modified, post_modified_gmt, post_content_filtered, post_parent, guid, menu_order, post_type, post_mime_type, comment_count )'. ' VALUES (NULL , 1, '.$dbw->Quote($data['created']).', '.$dbw->Quote($data['created']).', '.$dbw->Quote($post_content).', '.$dbw->Quote($data['title']).','.$dbw->Quote('').','.$dbw->Quote('publish').','.$dbw->Quote('open').','.$dbw->Quote('open').','.$dbw->Quote('').','.$dbw->Quote($data['alias']).','.$dbw->Quote('').','.$dbw->Quote('').','.$dbw->Quote($data['modified']).','.$dbw->Quote($data['modified']).','.$dbw->Quote('').',0,'.$dbw->Quote('http://localhost/wordpress/?p=').','.$dbw->Quote($data['ordering']).','.$dbw->Quote('post').','.$dbw->Quote('').',0 )' ); if (!$dbw->query()) { $app->enqueueMessage("error in db saving " , 'warning'); return false; } $queryw=$dbw->getQuery(true); $queryw->select('max(ID) AS id'); $queryw->from('#__posts'); $dbw->setQuery($queryw); $messagew=$dbw->loadObjectList(); $dbw->setQuery( 'UPDATE #__posts SET guid = concat(guid,'.$messagew[0]->id.') where ID='.$messagew[0]->id.'' ); if (!$dbw->query()) { $app->enqueueMessage("error in update db " , 'warning'); return false; } $dbw->setQuery( 'INSERT INTO #__term_relationships ( object_id, term_taxonomy_id, term_order)'. ' VALUES ('.$messagew[0]->id.' , 4,0 )' ); if (!$dbw->query()) { $app->enqueueMessage("error in db saving in #__term_relationships" , 'warning'); return false; } $app->enqueueMessage("Article Saved to Wordpress ", 'message'); } } |
Call the above saveWord() at the end of the save() function like this:
1 | $this->saveWord(); |
When You save a new article in joomla It would look like this:
You can check your new created joomla article in wordpress post.
- Tags:
Posts
Very rapidly this site will be famous among all blogging visitors, due to it’s fastidious articles
astrid broderie